What is Hibernate
Hibernate is an opensource, light weight pure Java object-relational mapping (ORM) and persistence framework that allows you to map plain old Java objects to relational database tables. The application that deal with complex and heavy weight business logic and contains additional services like security, transaction management and etc is called an enterprise application.
What is a framework?
A framework is reusable semi finished application that can be customized to develop a specific application.
Feature’s of hibernate
- Hibernate persists java objects into database (Instead of primitives)
- Hibernate generates efficient queries for java application to communicate with Database
- It provides fine-grained exception handling mechanism. In hibernate we only have Un-checked exceptions, so no need to write try, catch, or no need to write throws (In hibernate we have the translator which converts checked to Un-checked)
- It supports synchronization between in-memory java objects and relational records
- Hibernate provides implicit connection pooling mechanism
- Hibernate supports a special query language(HQL) which is Database vendor independent
- Hibernate has capability to generate primary keys automatically while we are storing the records into database
- Hibernate addresses the mismatches between java and database
- Hibernate provides automatic change detection
- Database objects (tables, views, procedures, cursors, functions … etc) name changes will not affect hibernate code
- Supports over 30 dialects
- Lazy loading concept is also included in hibernate so you can easily load objects on start up time
- Getting pagination in hibernate is quite simple.
- Hibernate Supports automatic versioning of rows
- Hibernate supports Inheritance, Associations, Collections
- Hibernate provides caching mechanism for efficient data retrieval
- Hibernate provides transactional capabilities that can work with both stand-alone or java Transaction API (JTA) implementations etc
- Hibernate supports annotations, apart from XML
Disadvantages of hibernate
- Since hibernate generates lots of SQL statements at runtime so it is slower than pure JDBC
- Hibernate is not much flexible in case of composite mapping. This is not disadvantage since understanding of composite mapping is complex
- Hibernate does not support some type of queries which are supported by JDBC
- Boilerplate code issue, actually we need to write same code in several files in the same application, but spring eliminated this
Hibernate Architecture
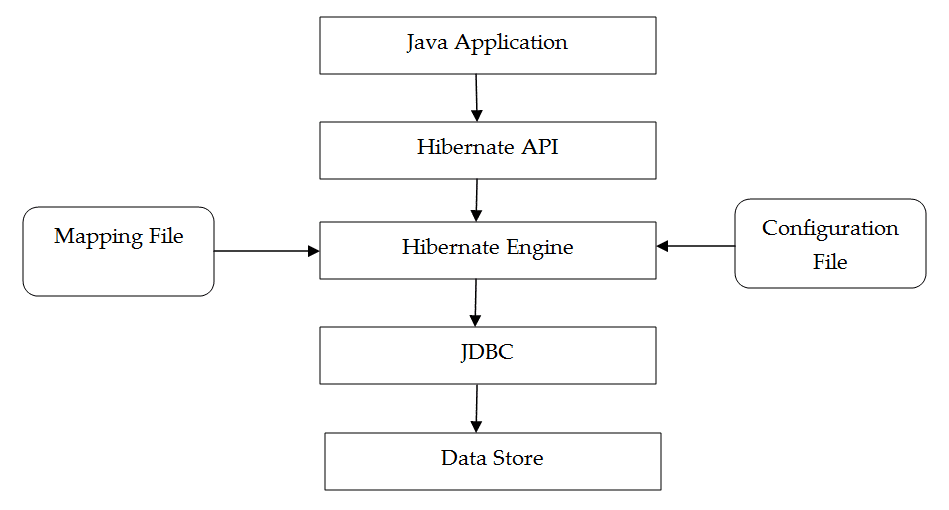
Java Application makes use of hibernate API methods calls to inform the persistent needs to hibernate. Then Hibernate engine generate JDBC code that corresponds to the underlying DB by using mapping file and configuration file information. We can also find the architecture diagrams as follows


Hibernate mapping file
- In this file hibernate application developer specify the mapping from entity class name to table name and entity properties names to table column names. i.e. mapping object oriented data to relational data is done in this file
- Standard name for this file is <domain-object-name.hbm.xml>Â
- In general, for each domain object we create one mapping file
Number of Entity classes = that many number of mapping xmls
- Mapping can be done using annotations also. If we use annotations for mapping then we no need to write mapping file.
- From hibernate 3.x version on wards it provides support for annotation, So mapping can be done in two ways
- XML
- Annotations
Syntax Of Mapping xml
<hibernate-mapping> <class name="POJO class name" table="table name in database"> <id name="variable name" column="column name in database" type="java/hibernate type" /> <property name="variable1 name" column="column name in database" type="java/hibernate type" /> <property name="variable2 name" column="column name in database" type="java/hibernate type" /> </class> </hibernate-mapping>
Hibernate configuration file
- It is an XML file in which database connection details (username, password, url, driver class name) and , Hibernate Properties(dialect, show-sql, second-level-cache … etc) and Mapping file name(s) are specified to the hibernate
- Hibernate uses this file to establish connection to the particular database serverÂ
- Standard name for this file is <hibernate.cfg.xml>
- We must create one configuration file for each database we are going to use, suppose if we want to connect with 2 databases, like Oracle, MySql, then we must create 2 configuration files.Â
No. of databases we are using = That many number of configuration files
- We can write this configuration in 2 ways …
- XML file
- Properties file(o1d style)
- We don’t have annotations to write configuration details. Actually in hibernate l.x, 2.x we defined this configuration by using .properties file, but from 3.x XML came into picture. XML files are always recommended to use.
Syntax Of Configuration XML
<hibernate-configuration> <session-factory> <!-- Related to the connection START --> <property name="connection.driver_class">Driver Class Name </property> <property name="connection.url">URL </property> <property name="connection.user">user </property> <property name="connection.password">password</property> <!-- Related to the connection END --> <!-- Related to hibernate properties START --> <property name="show_sql">true/false</property> <property name="dialet">Database dialet class</property> <property name="hbm2ddl.auto">create/update or what ever</property> <!-- Related to hibernate properties END--> <!-- Related to mapping START--> <mapping resource="hbm file 1 name .xml" / > <mapping resource="hbm file 2 name .xml" / > <!-- Related to the mapping END --> </session-factory> </hibernate-configuration>
Simple Hibernate Application Requirements
- Entity class
- Mapping file(Required if you are not using annotations)
- Configuration file
- DAO class (Where we write our logic to work with database)