Bridge Design Pattern
Bridge design pattern is one of the Structural design pattern. The Bridge Pattern is a structural design pattern that aids in the separation of an abstraction (high-level control logic) from its implementation (low-level functional logic), allowing both to be varied independently. This separation increases modularity and improves code maintainability.
We can better understand this pattern by using an example problem and its solution. Consider an example of a class hierarchy involving some vehicles and their transmission systems. We will go through this class hierarchy with and without the use of bridge pattern.
Scenario without bridge design pattern
- Base Class: Vehicle
- Sub Classes: Car, Truck
Next, if you want to incorporate different transmission systems for vehicles like Automatic
and Manual
, you will be required to create different combinations like automaticCar
, manualCar
, automaticTruck
, and manualTruck
. This is illustrated in the figure below:

As you try to introduce more types of vehicles like Bus
or motorcycle
, or more types of transmission systems like SemiAutomatic
, the number of classes will grow exponentially. This will make the system unwieldy and hard to maintain.
Bridge Pattern Solution
This problem occurs because we are trying to extend our Vehicle
class in multiple dimensions like vehicle type and vehicle transmission mode. This issue can be solved using the Bridge Pattern.
Bridge Pattern solves this problem by relating these two dimensions by using class composition instead of class inheritance. The classes will be separated into two different class hierarchies.
- Abstraction Layer (Vehicle Hierarchy):
- Base
Vehicle
class with a reference to aTransmission
object. - Subclasses like
Car
,Truck
,Bus
,Motorcycle
, etc., extendingVehicle
.
- Base
- Implementation Layer (Transmission Hierarchy):
Transmission
interface with methods likegearUp()
,gearDown()
.- Implementations like
Manual
,Automatic
, andSemiAutomatic
.
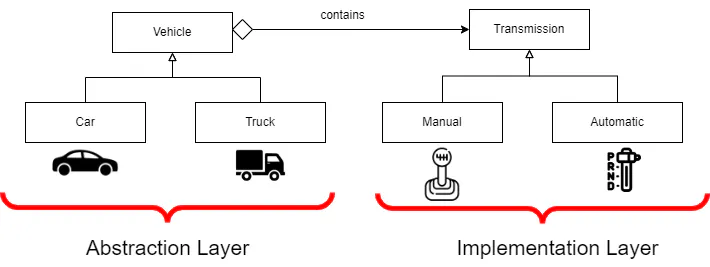
This separation allows you to introduce new vehicle types or transmission systems independently without affecting the other hierarchy, reducing the number of classes dramatically.
Abstraction and Implementation
Abstraction
The Bridge Pattern defines abstraction as the high-level layer with which the client interacts. It defines the abstract interface and keeps a reference to an implementation layer object.
In the Vehicle Example:
The class Vehicle is an abstract one. It is a representation of a generic vehicle that has a reference to an implementation layer Transmission object. Subclasses of Vehicle, such as Truck, Bus, and Car, are also included in it. Although they may have additional features or behaviors of their own, they nonetheless inherit high-level behaviors.
Implementation
The concrete implementation of the abstraction’s interface is provided by the implementation layer, which is independent of the abstraction. This layer carries out the low-level tasks.
In the Vehicle Example:
The implementation layer consists of the Transmission interface and its implementations (Manual, Automatic, SemiAutomatic). These classes offer particular functions for different transmission systems, like gear shifting.
Real-world Analogy
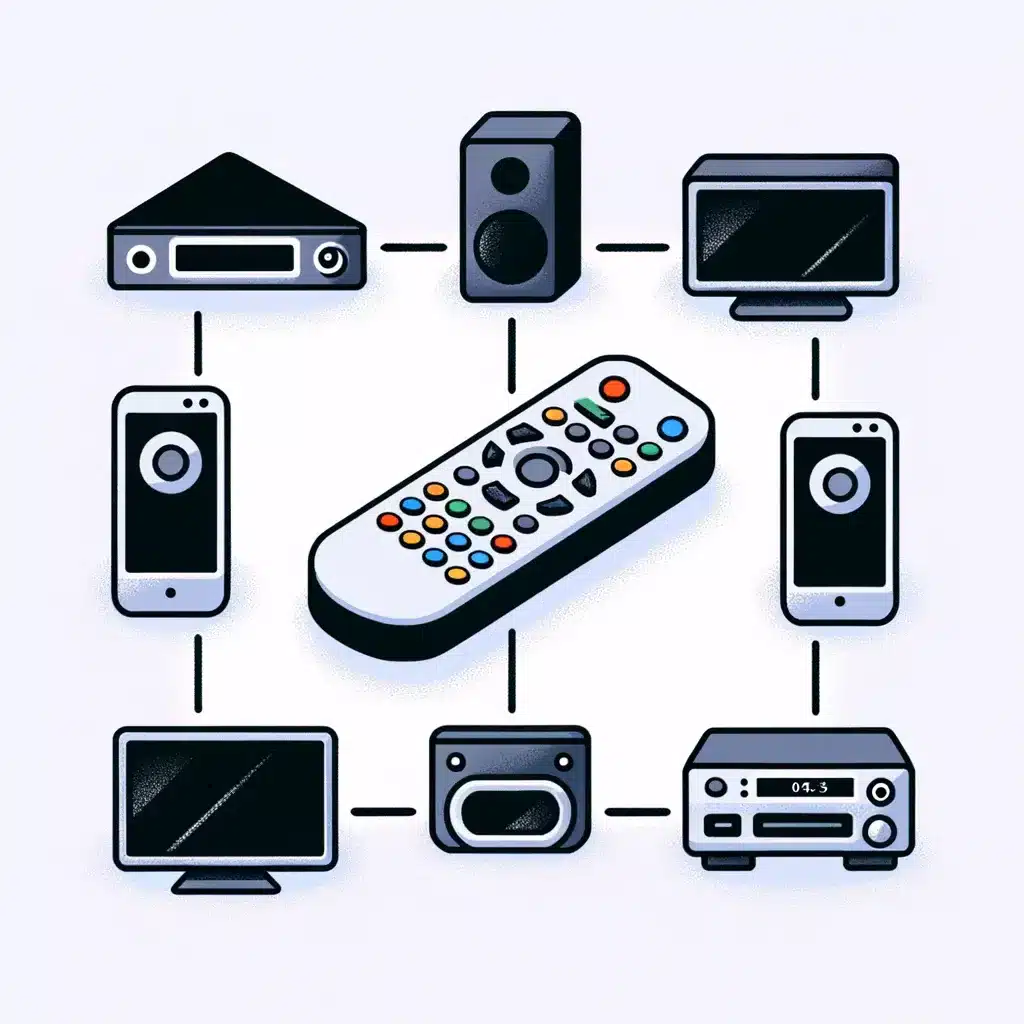
This illustration image shows a simple real-world example of a Bridge design Pattern by using a remote control system along with multiple electronic devices.
- In this illustration, the universal remote control system acts as an abstraction layer of the Bridge design Pattern. It provides some of the basic functionalities like play, stop, volume up or volume down, etc, but does not directly handle the specific operations of each device.
- The electronic devices acts as an Implementation layer where each device has its own particular way of handling different functionalities,
This configuration exemplifies the fundamental idea of the Bridge design Pattern: you may add new devices or modify current ones without changing the design of the remote control. The remote (abstraction) can operate many devices (implementations). Similar to this, the Bridge Pattern in software architecture enables an abstraction to communicate with different implementations, promoting both layers’ autonomous evolution and adaptability.
Structure of Bridge Design Pattern
- Abstraction: An abstract class or interface is usually used for this. It has an Implementor reference.
- Refined Abstraction: A concrete class that expands on the Abstraction is called
RefinedAbstraction
. - Implementor: A concrete implementor’s methods are defined by an interface.
- ConcreteImplementor: This is a concrete class that implements the interface
Implementor
. They have a realization (implementation) relationship with theImplementor
.
Implementation of Bridge Pattern
Looking back to our Vehicle class example, we will look at the implementation of that example in multiple programming languages. Are you excited to see the implementation? Let’s dive in!
Pseudocode
// Implementor
INTERFACE Transmission
METHOD applyGear()
// ConcreteImplementors
CLASS ManualTransmission IMPLEMENTS Transmission
METHOD applyGear()
// Implementation for manual transmission
CLASS AutomaticTransmission IMPLEMENTS Transmission
METHOD applyGear()
// Implementation for automatic transmission
// Abstraction
CLASS Vehicle
PROTECTED field transmission: Transmission
CONSTRUCTOR Vehicle(transmission)
this.transmission = transmission
METHOD applyTransmission()
// RefinedAbstraction
CLASS Car EXTENDS Vehicle
METHOD applyTransmission()
transmission.applyGear()
CLASS Truck EXTENDS Vehicle
METHOD applyTransmission()
transmission.applyGear()
// Client code
manual = NEW ManualTransmission()
car = NEW Car(manual)
car.applyTransmission()
In this pseudocode, we have followed the following steps:
- Define Implementor Interface:
Transmission
interface with a method likeapplyGear()
.
- Create Concrete Implementors:
- Implement the
Transmission
interface in classes likeManualTransmission
andAutomaticTransmission
.
- Implement the
- Establish Abstraction Class:
Vehicle
class containing a reference toTransmission
. It delegates transmission-related operations to theTransmission
object.
- Develop Refined Abstractions:
- Subclasses of
Vehicle
likeCar
andTruck
, which use theTransmission
interface methods.
- Subclasses of
- Client Interaction:
- The client combines a
Vehicle
with a specificTransmission
type and invokes methods, which are then delegated to theTransmission
object.
- The client combines a
Implementation
package com.ashok.designpatterns.bridge;
/**
*
* @author ashok.mariyala
*
*/
// Implementor
interface Transmission {
void applyGear();
}
package com.ashok.designpatterns.bridge;
/**
*
* @author ashok.mariyala
*
*/
// ConcreteImplementors
class ManualTransmission implements Transmission {
public void applyGear() {
System.out.println("Manual transmission applied.");
}
}
class AutomaticTransmission implements Transmission {
public void applyGear() {
System.out.println("Automatic transmission applied.");
}
}
package com.ashok.designpatterns.bridge;
/**
*
* @author ashok.mariyala
*
*/
// Abstraction
abstract class Vehicle {
protected Transmission transmission;
public Vehicle(Transmission transmission) {
this.transmission = transmission;
}
abstract void applyTransmission();
}
package com.ashok.designpatterns.bridge;
/**
*
* @author ashok.mariyala
*
*/
// RefinedAbstraction
class Car extends Vehicle {
public Car(Transmission transmission) {
super(transmission);
}
void applyTransmission() {
transmission.applyGear();
}
}
package com.ashok.designpatterns.bridge;
/**
*
* @author ashok.mariyala
*
*/
// RefinedAbstraction
class Truck extends Vehicle {
public Truck(Transmission transmission) {
super(transmission);
}
void applyTransmission() {
transmission.applyGear();
}
}
package com.ashok.designpatterns.bridge;
/**
*
* @author ashok.mariyala
*
*/
// Client code
public class Solution {
public static void main(String[] args) {
Transmission manual = new ManualTransmission();
Vehicle car = new Car(manual);
car.applyTransmission();
}
}
Application of Bridge Design Pattern
We have understood the concept of bridge design pattern, but, when to use it? Let’s look at some of the scenarios where bridge pattern can help us out.
- When to Expect Variations Between Implementations and Abstractions: The Bridge design Pattern is a suitable option if you know that the low-level logics (implementation) and the high-level logic (abstraction) are likely to change or extend separately.
- In Order to Prevent Permanent Binding Between Implementation and Abstraction: when you wish to prevent a permanent coupling between an abstraction and its implementations and the abstraction can work with many other implementations.
- To Keep Implementation Details Private from Clients: It’s advantageous to keep certain implementation details secret from clients. Just what is required is exposed by the abstraction layer; the implementation layer is responsible for the remaining information.
- Refactoring a Monolithic Class Hierarchy: The Bridge design Pattern can assist in dividing a class hierarchy into discrete functional groups if it comprises multiple distinct functionality.
- Platform independence: Bridge design Pattern is helpful when creating a system that can operate on several different platforms and allows you to expand or change the portions that are platform-specific.
The Bridge Design Pattern is a powerful technique for managing code complexity and ensuring flexibility in evolving systems. It allows developers to vary implementations independently from the abstractions that use them.
That’s all about the Bridge Design Pattern. If you have any queries or feedback, please write us email at contact@waytoeasylearn.com. Enjoy learning, Enjoy Design patterns.!!