LinkedHashMap Class
In this tutorial, we are going to discuss about LinkedHashMap class in java. LinkedHashMap will keep the order in which the elements are inserted into it. If you will be adding and removing elements a lot, it will be better to use HashMap, because LinkedHashMap will be slower to do those operations. But, you can iterate faster using LinkedHashMap.
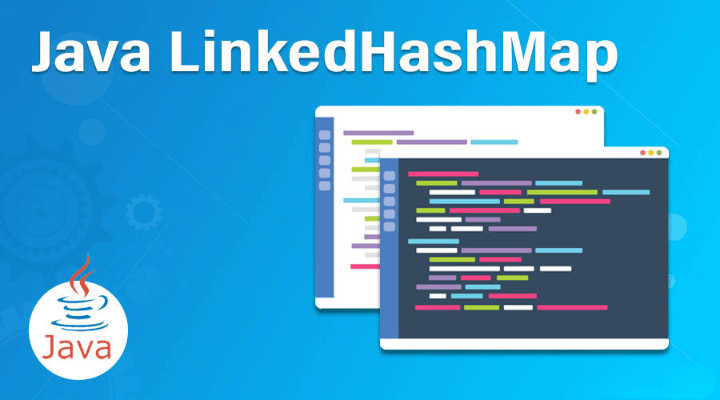
So, if you will be iterating heavily, it may be a good idea to use this. It is exactly same as HashMap except the following differences

package com.ashok.collections;
import java.util.Collection;
import java.util.LinkedHashMap ;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
/**
*
* @author ashok.mariyala
*
*/
public class MyHashMap {
public static void main(String[] args) {
LinkedHashMap hMap = new LinkedHashMap ();
hMap.put("Ashok", 500);
hMap.put("Vinod", 800);
hMap.put("Dillesh", 700);
hMap.put("Naresh", 500);
System.out.println(hMap);
System.out.println(hMap.put("Ashok", 1000));
Set s = hMap.keySet();
System.out.println(s);
Collection c = hMap.values();
System.out.println(c);
Set s1 = hMap.entrySet();
System.out.println(s1);
Iterator itr = s1.iterator();
while (itr.hasNext()) {
Map.Entry m1 = (Map.Entry) itr.next();
System.out.println(m1.getKey() + "......" + m1.getValue());
if (m1.getKey().equals("Ashok")) {
m1.setValue(1500);
}
}
System.out.println(hMap);
}
}
Output
{Ashok=500, Vinod=800, Dillesh=700, Naresh=500} 500 [Ashok, Vinod, Dillesh, Naresh] [1000, 800, 700, 500] [Ashok=1000, Vinod=800, Dillesh=700, Naresh=500] Ashok......1000 Vinod......800 Dillesh......700 Naresh......500 {Ashok=1500, Vinod=800, Dillesh=700, Naresh=500}
Key Features of LinkedHashMap class
- Preserves Insertion Order: Unlike
HashMap
,LinkedHashMap
class maintains the order in which elements were inserted into the map. Iterating over the map will produce the elements in the same order they were inserted. - Hash Table with Linked List: Internally,
LinkedHashMap
class uses a combination of a hash table and a doubly-linked list to store key-value pairs. The linked list maintains the insertion order of elements, while the hash table provides fast lookup and retrieval. - Access Order:
LinkedHashMap
provides a constructor that allows you to create a map that maintains access order instead of insertion order. In access-order mode, the map is reordered based on the most recent access (either by key or by value). - Not Synchronized: Like
HashMap
,LinkedHashMap
is not synchronized, meaning it is not thread-safe for concurrent access by multiple threads. If thread safety is required, synchronization must be handled externally. - Iterating Over Entries:
LinkedHashMap
provides methods to iterate over its entries in insertion order or access order.
That’s all about the LinkedHashMap class in java. If you have any queries or feedback, please write us email at contact@waytoeasylearn.com. Enjoy learning, Enjoy Java.!!
LinkedHashMap Class