Variables in GO
In this tutorial, we are going to discuss variables in the Go language. There are various syntaxes to declare variables in Go. Let’s look at them one by one.
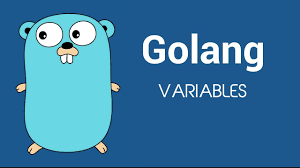
A variable is the name of a memory location. That memory location may store a value of any type. So each variable has a type associated with it that determines the size and range of that variable and the operations defined on that variable.
Declaring a variable
The keyword var is used for declaring variables. To declare a single variable, you can use the following syntax.
var variableName dataType
E.g
package main
import "fmt"
func main() {
var name string // variable declaration
fmt.Println("My name is", name)
}
The statement var name string declares a variable named name of the type string. We have not assigned any value to this variable.
If a variable is not assigned any value, Go automatically initializes it with the zero value of the variable’s type. In this case, the name is assigned the value emptystring (“”), which is the zero value of the string. If you run this program, you can see the below output.
My name is
Naming Conventions
- A variable name can only start with a letter or an underscore.
- It can be followed by any number of letters, numbers or underscores after that
- Go is case sensitive so uppercase and lowercase letters are treated differently.
- The variable name cannot be any keyword name in Go
- There is no limit on the length of the variable name.
- But it is advisable to have the variable name of optimum length.
Zero values
Variables declared without an explicit initial value are given their zero value.
The zero value is:
- 0 for numeric types,
- “” (the empty string) for strings and
- false for the boolean type.
A variable can be assigned to any value of its type. In the above program, we can set the name of any string value.
package main
import "fmt"
func main() {
var name string // variable declaration
fmt.Println("My name is", name)
name = "Ashok" //assignment
fmt.Println("My name is", name)
name = "Ashok Kumar" //assignment
fmt.Println("My updated name is", name)
}
Output
My name is
My name is Ashok
My updated name is Ashok Kumar
Declaring a variable with an initial value
A variable can also be provided an initial value when it is declared. The following is the syntax to declare a variable with an initial value.
var variableName dataType = initialValue
E.g
package main
import "fmt"
func main() {
var name string = "Ashok Kumar"
fmt.Println("My name is", name)
}
Output
My name is Ashok Kumar
Here are some essential things to know about Golang variables.
- Golang is a statically typed language, which means that when Golang variables are declared, they either explicitly or implicitly assigned a type even before your program runs.
2. Golang requires that every variable you declare inside the main() function get used somewhere in your program.
3. You can assign a new value to an existing variable, but the value needs to be of the same type.
4. A variable declared within brace brackets {} may be accessed anywhere within the block. The opening curly brace { introduces a new scope that ends with a closing brace }. Inner blocks can access variables within outer blocks. Outer blocks cannot access variables within inner blocks.
Variable Declaration Omit Types
If a variable has an initial value, Go will automatically infer the type of that variable using that initial value. This is called Type inference.
Hence if a variable has an initial value, We can remove the type in the variable declaration. If the variable is declared using the following syntax
var name = initialvalue
Go will automatically infer the type of that variable from the initial value.
E.g
package main
import "fmt"
func main() {
var name = "Ashok Kumar"
var age = 29
fmt.Println("My name is", name, "and age is" , age)
}
Output
My name is Ashok Kumar and age is 29
Short Variable Declaration in Golang
Go also provides another concise way to declare variables. This is known as short hand declaration and it uses := operator. There is no need to use the var keyword or declare the variable type.
E.g
package main
import "fmt"
func main() {
name := "Ashok Kumar"
fmt.Println("My name is", name)
}
Output
My name is Ashok Kumar
Declare Multiple Variables
Golang allows you to assign values to multiple variables in one line.
E.g
package main
import "fmt"
func main() {
var fname, lname string = "Ashok Kumar", "Mariyala"
fmt.Println("My first name is", fname)
fmt.Println("My last name is", lname)
}
Output
My first name is Ashok Kumar
My last name is Mariyala
We can remove the type if the variables have an initial value. Since the above program has initial values for variables, we can remove the string type.
E.g
package main
import "fmt"
func main() {
var fname, lname = "Ashok Kumar", "Mariyala"
fmt.Println("My first name is", fname)
fmt.Println("My last name is", lname)
}
Output
My first name is Ashok Kumar
My last name is Mariyala
There might be cases where we want to declare variables belonging to different types in a single statement. The syntax for doing that is
var (
name1 = initialvalue1
name2 = initialvalue2
name3 = initialvalue3
)
E.g
package main
import "fmt"
func main() {
var (
name = "Ashok Kumar"
age = 29
salary int
)
fmt.Println("My name is", name, ", age is", age, "and salary is", salary)
}
Here we declare a variable name of type string, age, and salary of type int.
Note
- An unused variable will be reported as a compiler error. Go compiler doesn’t allow any unused variable. This is an optimization in GO. The same is applicable for constant too, as we will see later.
- A variable declared within an inner scope having the same name as variable declared in the outer scope will shadow the variable in the outer scope.
- Once initialized with a particular type, a variable cannot be assigned a value of a different type later. This is applicable for shorthand declaration as well.
That’s all about the Variables in Go language. If you have any queries or feedback, please write us email at contact@waytoeasylearn.com. Enjoy learning, Enjoy Go language.!!