BufferedWriter Class
In this tutorial, we are going to discuss about BufferedWriter Class in java. This can be used for writing character data to the file.
The BufferedWriter
class in Java, found in the java.io
package, is used to write character data to a destination, such as a file, with buffering for improved performance. It wraps another Writer
, such as a FileWriter
, and buffers the output before writing it to the underlying destination.
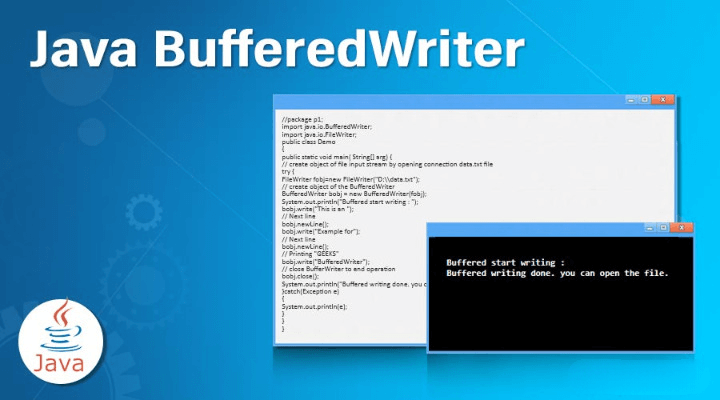
Constructors
BufferedWriter bw = new BufferedWriter(writer w);
BufferedWriter bw = new BufferedWriter(writer r, int size);
BufferedWriter never communicates directly with the file. It should Communicate through some writer object only.
BufferedWriter bw = new BufferedWriter(“ashok.txt”); // Invalid
BufferedWriter bw = new BufferedWriter(new File(“ashok.txt”)); // Invalid
BufferedWriter bw = new BufferedWriter(new FileWriter(“ashok.txt”)); // Valid
BufferedWriter bw = new BufferedWriter(new BufferedWriter(new FileWriter(“ashok.txt”))); // Valid
Important methods
void write(int ch) thorows IOException
void write(String s) throws IOException
void write(char[] ch) throws IOException
void newLine()
void flush()
void close()
E.g
package com.ashok.files;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
public class MyFile {
public static void main(String arg[]) throws Exception {
File f = new File("ashok.txt");
System.out.println(f.exists());
FileWriter fw = new FileWriter(f);
BufferedWriter bw = new BufferedWriter(fw);
bw.write(97);
bw.newLine();
char [] ch = {'a','b','c','d'};
bw.write(ch);
bw.newLine();
bw.write("Ashok");
bw.newLine();
bw.write("Waytoeasylearn");
bw.flush();
bw.close();
}
}
Output
true
In ashok.txt
a
abcd
Ashok
Waytoeasylearn
Note
When ever we are closing BufferedWriter, automatically underlying FileWriter object will be closed.
That’s all about the BufferedWriter Class in java. If you have any queries or feedback, please write us email at contact@waytoeasylearn.com. Enjoy learning, Enjoy Java.!!