for range loop in Go
In this tutorial, we are going to discuss for range loop in Go language. When it comes to loop, go language has:
- for loop
- for-range loop
We saw for loop in the earlier tutorial. In this tutorial, we will be learning about the for range loop.
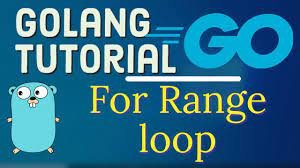
The for range loop is used to iterate over different collection data structures in go language such as
- array or slice
- string
- maps
- channel
Let’s see some examples now
for-range loop for array/slice
Following is the syntax of for range when used with array/slice.
for index, value := range array / slice {
//Do something with index and value
}
This is how for range loop works in the case of array/slice. It iterates over the given array/slice starting from index zero, and the body of the for range loop is executed for every value present at the index. Both index and value are optional in for range when using with array/slice.
The following example shows how to use a for range loop for a slice
package main
import "fmt"
func main() {
names := []string{"Ashok", "Rama", "Seetha", "Lakshmana"}
//With index and value
fmt.Println("Both Index and Value")
for i, name := range names {
fmt.Printf("Index: %d Value: %s\n", i, name)
}
//Only value
fmt.Println("\nOnly value")
for _, name := range names {
fmt.Printf("Value: %s\n", name)
}
//Only index
fmt.Println("\nOnly Index")
for i := range names {
fmt.Printf("Index: %d\n", i)
}
//Without index and value. Just print array values
fmt.Println("\nWithout Index and Value")
i := 0
for range names {
fmt.Printf("Index: %d Value: %s\n", i, names[i])
i++
}
}
Output
Both Index and Value
Index: 0 Value: Ashok
Index: 1 Value: Rama
Index: 2 Value: Seetha
Index: 3 Value: Lakshmana
Only value
Value: Ashok
Value: Rama
Value: Seetha
Value: Lakshmana
Only Index
Index: 0
Index: 1
Index: 2
Index: 3
Without Index and Value
Index: 0 Value: Ashok
Index: 1 Value: Rama
Index: 2 Value: Seetha
Index: 3 Value: Lakshmana
for range loop with a string
In Go language, the string is a sequence of bytes. A string literal represents a UTF-8 sequence of bytes. In UTF-8, ASCII characters are single-byte corresponding to the first 128 Unicode characters. All other characters are between 1 -4 bytes.
Now let’s see a code example
package main
import "fmt"
func main() {
name := "Ashok"
//With index and value
fmt.Println("Both Index and Value")
for i, letter := range name {
fmt.Printf("Start Index: %d Value:%s\n", i, string(letter))
}
//Only value
fmt.Println("\nOnly value")
for _, letter := range name {
fmt.Printf("Value:%s\n", string(letter))
}
//Only index
fmt.Println("\nOnly Index")
for i := range name {
fmt.Printf("Start Index: %d\n", i)
}
}
Output
Both Index and Value
Start Index: 0 Value:A
Start Index: 1 Value:s
Start Index: 2 Value:h
Start Index: 3 Value:o
Start Index: 4 Value:k
Only value
Value:A
Value:s
Value:h
Value:o
Value:k
Only Index
Start Index: 0
Start Index: 1
Start Index: 2
Start Index: 3
Start Index: 4
for range loop with a map
In the case of the map, for range iterates over key and values of a map. Below is the syntax for the for range when using with a map
for key, value := range map {
//Do something with key and value
}
A point to be noted is that both key and value are optional to be used while using for range with maps. Let’s see a simple code example.
package main
import "fmt"
func main() {
capitalCities := map[string]string{
"India": "Delhi",
"England": "London",
"USA": "Washington DC",
}
//Iterating over all keys and values
fmt.Println("Both Key and Value")
for k, v := range capitalCities {
fmt.Printf("key :%s, value: %s\n", k, v)
}
//Iterating over only keys
fmt.Println("\nOnly keys")
for k := range capitalCities {
fmt.Printf("key :%s\n", k)
}
//Iterating over only values
fmt.Println("\nOnly values")
for _, v := range capitalCities {
fmt.Printf("value :%s\n", v)
}
}
Output
Both Key and Value
key :England, value: London
key :USA, value: Washington DC
key :India, value: Delhi
Only keys
key :India
key :England
key :USA
Only values
value :Delhi
value :London
value :Washington DC
for-range loop with a channel
for range loop works differently too for a channel. An index doesn’t make any sense for a channel as the channel is similar to a pipeline where values enter from one and exit from the other end.
So in the case of the channel, the for-range loop will iterate over values currently present in the channel. After it has iterated over all the values currently present (if any), the for-range loop will not exit but instead wait for the next value that might be pushed to the channel, and it will exit only when the channel is closed.
Following is the syntax when using for range with channel
for value := range channel {
//Do something value
}
Let’s see a code example
package main
import "fmt"
func main() {
channel := make(chan string)
go pushToChannel(channel)
for value := range channel {
fmt.Println(value)
}
}
func pushToChannel(channel chan<- string) {
channel <- "Ashok"
channel <- "Rama"
channel <- "Seetha"
channel <- "Lakshmana"
close(channel)
}
Output
Ashok
Rama
Seetha
Lakshmana
That’s all about the for range loop in Go language. If you have any queries or feedback, please write us email at contact@waytoeasylearn.com. Enjoy learning, Enjoy Go language.!!