AOP Terminology
In this tutorial, we are going to discuss AOP terminology in the spring framework. In general, AOP will use the following terminologies to implement AOP-based implementation.
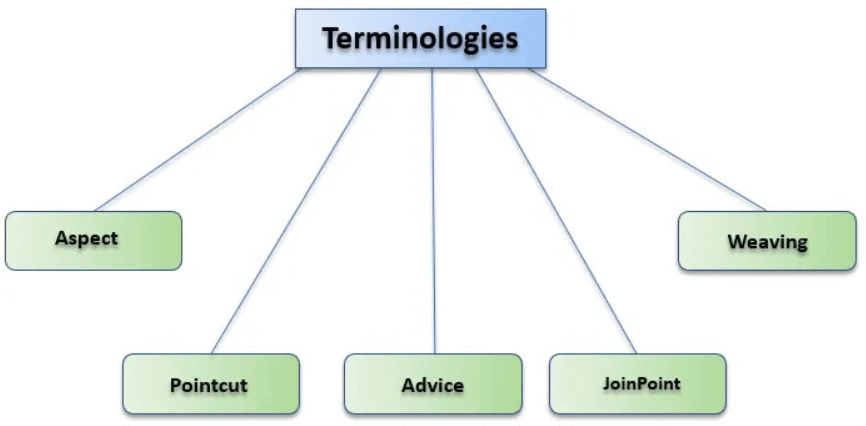
- Aspect
- Pointcut
- Advice
- JoinPoint
- Weaving
It would be not easy to understand the above terminologies theoretically. So, let us witness a practical example of using Spring AOP and how the terminologies are interconnected.
package com.ashok.spring.aop;
import org.springframework.stereotype.Service;
/**
*
* @author ashok.mariyala
*
*/
@Service
public class TransactionService {
public void loggingService() {
// Business Logic
}
public void emailService() {
// Business Logic
}
public void messagingService() {
// Business Logic
}
}
package com.ashok.spring.aop;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.context.annotation.Configuration;
/**
*
* @author ashok.mariyala
*
*/
@Aspect
@Configuration
public class AspectConfiguration {
private static final Logger LOG = LoggerFactory.getLogger(AspectConfiguration.class.getName());
@Before("execution(* com.ashok.spring.aop.*.*(..))") // Pointcut
public void before(JoinPoint joinPoint) {
// Advice
LOG.info("Started Executing "+joinPoint.getSignature().getName());
}
@After("execution(* com.ashok.spring.aop.*.*(..))") // Pointcut
public void after(JoinPoint joinPoint) {
// Advice
LOG.info("Completed Executing "+joinPoint.getSignature().getName());
}
}
From the above code snippet, Logging Management needs to be achieved during the start and completion for every method in TransactionService.
If we need to write loggers during the start and end of each method in the business layer, that would be a tedious task. So, we are assigning the logging activities to be taken care of by Spring AOP. So, we are making use of Spring AOP to centralize the Logging Management.
Aspect
- Aspect is nothing but the concern or the functionality that you are trying to implement generally or centrally.
- From the above example, the aspect is Logging Management.
- Some of the aspects used across the industry are Logging Management, Transaction Management, Exception Handling, Performance Metrics, Authentication, Security, etc.,
- In Simple terms, aspect is the functionality that you are trying to achieve through Spring AOP.
Pointcut
A pointcut is nothing but a kind of regular expression that specifies, what are the method calls that need to be intercepted. From the code snippet in AspectConfiguration,
@Before("execution(* com.ashok.spring.aop.*.*(..))")
@After("execution(* com.ashok.spring.aop.*.*(..))")
The Regular expression denotes all the methods (using .) in package com.ashok.spring.aop needs to be intercepted. Also, @Before specifies that logging needs to happen once the control enters into the method and @After specifies that logging needs to occur once the control exits the method.
Advice
Advice is nothing but what is the action that needs to be done when a Pointcut is met. From the code snippet in AspectConfiguration,
LOG.info("Started Executing " + joinPoint.getSignature().getName());
So here the Advice is to start logging the information along with the method name when a Pointcut is met.
JoinPoints
A joinpoint is a specific point in the application such as method execution, exception handling, changing object variable values, etc. In Spring AOP, a joinpoint is always the execution of a method.
Weaving
Weaving is nothing but when a Pointcut is met, the corresponding method will get executed. From the code snippet, Line no. 21 & 22 – When Pointcut is met, it makes sure to execute before() method. This process of binding the Pointcut with the method is referred to as Weaving.
Advantages of Spring AOP
Some of the advantages of Spring AOP are,
- AOP is one of the key components of the Spring Framework. It is important to note that Spring IoC Containers is not dependent on AOP. So, it provides the advantage to developers to whether to use AOP or not.
- As an implementation of cross-cutting concerns, functionalities such as Logging, Notification Management, Authentication, Security, Transaction Management can be kept in a centralized manner and can be used across multiple places in the application.
- As AOP is implemented using Java, there is no need for special compilation units or class loaders.
- As the cross-cutting concerns are centralized, Boilerplate coding is reduced.
- It becomes easy for the developers to maintain the system.
- Spring AOP provides XML based configuration as well as advanced Java Annotation configuration.