Packages
In this tutorial, we are going to discuss about packages in java. In Java, packages are used to organize classes and interfaces into namespaces, providing a way to group related code together and avoid naming conflicts.
Packages also help in maintaining the structure of large projects, improving code reusability, and facilitating modular development.
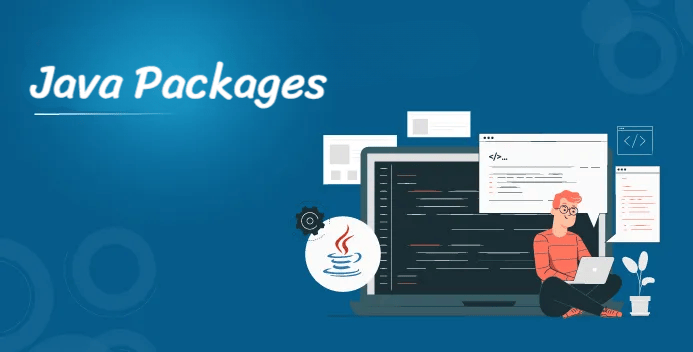
Here’s an overview of Java packages:
Package is an encapsulation mechanism to group related classes and interfaces into a single module. The main purpose of packages are
- To resolve naming conflicts
- To provide security to the classes and interfaces. So that outside person can’t access directly.
- It improves modularity of the application.
There is one universally accepted convention to name packages i.e., to use internet domain name in reverse.
com.icicibank.loan.houseingloan.Account
package com.ashok.helloworld;
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello World");
}
}
javac HelloWorld.java
The generated class file will be placed in current working directory.
javac -d . HelloWorld.java
-d : Destination to place generated class files
. : Current working directory
Generated class file will be placed into corresponding package structure.
- If the specified package structure is not already available then this command itself will create that package structure.
- As the destination we can use any valid directoryPackage Declaration: Each Java source file begins with a package declaration that specifies the package to which the class or interface belongs. This declaration is optional, but if present, it must be the first non-comment line in the file.
E.g
javac -d c: HelloWorld.java
If the specified destination is not available then we will get compilation error
E.g
javac -d z: HelloWorld.java
Here z: is not already available then we will get compile time error
Conclusions
In any java program there should be only at most 1 package statement. If we are taking more than one package statement we will get compile time error.
E.g
package pack1;
package pack2;
class A {
}
C.E: class, interface or enum expected
In any java program the first non comment statement should be package statement if it is available.
E.g
import java.util.*;
package com.ashok;
class A {
}
C.E: class, interface or enum expected
Package Declaration
Each Java source file begins with a package declaration that specifies the package to which the class or interface belongs. This declaration is optional, but if present, it must be the first non-comment line in the file.
package com.ashok.myproject;
Package Naming Convention
Packages are typically named using a reverse domain name convention to ensure uniqueness and avoid conflicts. For example, if your domain name is example.com
, you might create packages under com.ashok
:
com.ashok.myproject
com.ashok.utilities
com.ashok.model
Subpackages
Packages can have subpackages, allowing for further organization of code. Subpackages are separated by dots in the package name.
package com.ashok.myproject.utilities;
Access Control
Java provides four access levels for classes, interfaces, fields, and methods within packages:
public
: Accessible from any other class.protected
: Accessible from classes in the same package or subclasses.Default (no modifier)
: Accessible only within the same package.private
: Accessible only within the same class.
Importing Packages
To use classes or interfaces from other packages within your code, you need to import them using the import
statement. This statement tells the compiler where to find the classes you’re referencing.
import java.util.List;
import com.ashok.utilities.UtilityClass;
Java Standard Library Packages
Java Standard Library provides a vast collection of packages covering various functionalities. Some commonly used packages include:
java.lang
: Provides fundamental classes and interfaces, automatically imported in every Java program.java.util
: Contains utility classes and data structures like collections, arrays, and dates.java.io
: Offers classes for input and output operations, including file handling.java.net
: Provides classes for networking operations, such as working with URLs and sockets.java.awt
,javax.swing
: For creating graphical user interfaces (GUI).
Creating Custom Packages
To create your own package, you simply create a directory structure that mirrors the package name and place your Java files within that structure. For example, if you’re creating a package com.ashok.myproject
, you would create a directory structure like this:
com
└── ashok
└── myproject
└── MyClass.java
Then, you would add the package declaration to MyClass.java
:
package com.ashok.myproject;
By organizing classes and interfaces into packages, you can better manage your Java projects, improve code readability, and maintain scalability and reusability.
That’s all about Packages in Java. If you have any queries or feedback, please write us at contact@waytoeasylearn.com. Enjoy learning, Enjoy Java.!!