Customized Serialization
In this tutorial, we are going to discuss about Customized Serialization in Java. During default Serialization there may be a chance of loss of information because of transient variables.
Customized serialization in Java allows you to have fine-grained control over the serialization and deserialization process by providing custom logic for serializing and deserializing objects. This can be useful when you need to handle special cases, such as transient fields, derived fields, or non-serializable fields.
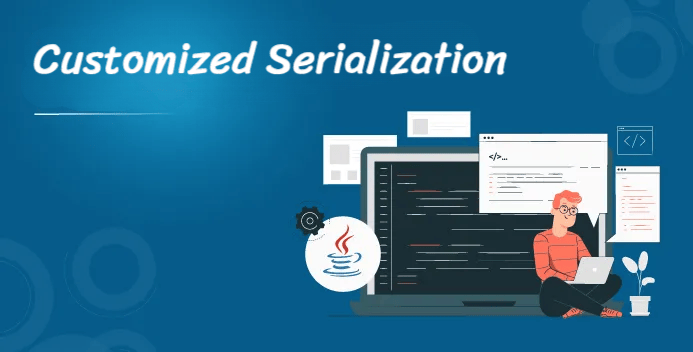
package com.ashok.files;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
/**
*
* @author ashok.mariyala
*
*/
class Dog implements Serializable {
Cat c = new Cat();
}
class Cat {
String name = "Welcome to Waytoeasylearn.com";
}
public class MySerialization {
public static void main(String arg[]) throws Exception {
Dog d = new Dog();
FileOutputStream fos = new FileOutputStream("ashok.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(d);
FileInputStream fis = new FileInputStream("ashok.ser");
ObjectInputStream ois = new ObjectInputStream(fis);
Dog d1 = (Dog) ois.readObject();
System.out.println(d1.c.r.j);
}
}
Output
Exception in thread "main" java.io.NotSerializableException: com.ashok.files.Cat
Here we ensure that the class of the object you want to serialize implements the java.io.Serializable
interface. We can implement customized Serialization by using the following two methods.
private void writeObject(OutputStream os)throws Exception {
..........
..........
}
This method will be executed automatically by the JVM at the time of Serialization.
private void readObject(InputStream is)throws Exception {
..........
..........
}
This method will be executed automatically by the JVM at the time of deSerialization.
package com.ashok.files;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
/**
*
* @author ashok.mariyala
*
*/
class Dog implements Serializable {
transient Cat c = new Cat();
private void writeObject(ObjectOutputStream os) throws IOException {
int x = c.j;
os.writeInt(x);
}
private void readObject(ObjectInputStream is) throws IOException, ClassNotFoundException {
is.defaultReadObject();
int k = is.readInt();
c = new Cat();
c.j = k;
}
}
class Cat {
int j = 100;
}
class SerializeDemo {
public static void main(String arg[]) throws Exception {
Dog d = new Dog();
System.out.println("Before Serialization:" + d.c.j);
FileOutputStream fos = new FileOutputStream("ashok.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(d);
FileInputStream fis = new FileInputStream("ashok.ser");
ObjectInputStream ois = new ObjectInputStream(fis);
Dog d1 = (Dog) ois.readObject();
System.out.println(d1.c.j);
}
}
Output
100
That’s all about the Customized Serialization in java. If you have any queries or feedback, please write us email at contact@waytoeasylearn.com. Enjoy learning, Enjoy Java.!!