Dead lock
In this tutorial, we are going to discuss about dead lock in java. A deadlock in multithreading occurs when two or more threads are blocked indefinitely, each waiting for the other to release a resource that it needs, resulting in a circular waiting condition. Deadlocks can significantly impact the stability and performance of a multithreaded application.
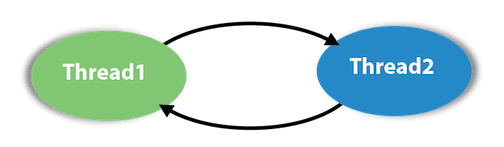
- If two Threads are waiting for each other forever(without end) such type of situation(infinite waiting) is called dead lock.
- There are no resolution techniques for dead lock but several prevention(avoidance) techniques are possible.
- Synchronized keyword is the cause for deadlock hence whenever we are using synchronized keyword we have to take special care.
Here’s a typical scenario that leads to a deadlock:
- Acquiring Multiple Locks: Two or more threads acquire locks on resources in a different order.
- Circular Waiting: Each thread holds a lock that the other thread needs, creating a circular dependency.
- Indefinite Blocking: Since each thread is waiting for the other to release the lock it holds, none of the threads can proceed, leading to a deadlock.
public class DeadlockExample {
private static final Object resource1 = new Object();
private static final Object resource2 = new Object();
public static void main(String[] args) {
Thread thread1 = new Thread(() -> {
synchronized (resource1) {
System.out.println("Thread 1: Acquired resource1 lock");
try {
Thread.sleep(100); // Simulate some work
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Thread 1: Waiting for resource2 lock");
synchronized (resource2) {
System.out.println("Thread 1: Acquired resource2 lock");
}
}
});
Thread thread2 = new Thread(() -> {
synchronized (resource2) {
System.out.println("Thread 2: Acquired resource2 lock");
try {
Thread.sleep(100); // Simulate some work
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Thread 2: Waiting for resource1 lock");
synchronized (resource1) {
System.out.println("Thread 2: Acquired resource1 lock");
}
}
});
thread1.start();
thread2.start();
}
}
In this example, thread1
acquires resource1
and then attempts to acquire resource2
, while thread2
acquires resource2
and then attempts to acquire resource1
. If these threads are executed concurrently, a deadlock occurs because each thread is holding a lock that the other thread needs.
To prevent deadlocks, it’s crucial to follow best practices for thread synchronization, such as acquiring locks in a consistent order and using mechanisms like timeouts and deadlock detection algorithms. Additionally, minimizing the use of locks where possible and using higher-level concurrency utilities provided by Java’s java.util.concurrent
package can help reduce the likelihood of deadlocks.
That’s all about dead lock in java. If you have any queries or feedback, please write us at contact@waytoeasylearn.com. Enjoy learning, Enjoy Java.!!