Template Method Pattern
The template method pattern is a behavioral design pattern that defines the skeleton of an algorithm in the super class but allows sub classes to change specific steps without changing the algorithm’s structure. Put differently, it acts as an algorithm’s template, letting subclasses take over specific steps while maintaining its general structure.
The pattern adheres to the “Don’t call us, we’ll call you” principle, in which the subclasses are assigned specific tasks by the superclass, which contains the fixed steps of the algorithm.
Consider creating a program that can provide reports in several formats, including CSV, HTML, and PDF. The procedures for gathering data, processing data, and producing reports are the same for all report types. The details of every step, however, differ based on the kind of report. Implementing each report type independently results in code redundancy, particularly in processes that are shared by all report kinds.

The Template Method design handles this by encapsulating the algorithm’s skeleton in a method and delegating some steps to subclasses. The image above illustrates that an abstract base class ReportGenerator
with a template function generateReport()
can be created. The report creation process is defined by this method; however, the subclasses are responsible for carrying out specific tasks such as data collecting, processing, and formatting.
- Data Collection: An abstract approach for gathering information. Depending on the type of report, this may include reading a file, performing an API call, or querying a database.
- Data processing: An additional abstract technique that allows the data to be processed as needed for each sort of report.
- Formatting: A procedure unique to each kind of report that converts data into the format of choice (PDF, HTML, CSV).
This way, the common structure of the report generation process is defined in the base class while allowing flexibility in implementing each step. The result is a more organized and less redundant codebase, where common functionalities are centralized, and sub classes handle specific details.
Real-World Example
A real-world example of the Template Method pattern can be found in the process of making different types of beverages, such as coffee and tea. The overall process of preparing a hot beverage involves common steps like boiling water, brewing, and serving. However, the specific steps for brewing and serving differ between coffee and tea.
In this scenario:
- The abstract class represents the generic process of making a hot beverage. It includes a template method that outlines the overall steps like boiling water, brewing, and serving.
- The concrete classes, Coffee and Tea, extend the abstract class. They provide specific implementations for the steps like brewing and serving, which are unique to each type of beverage.
The illustration below shows two parts, one for coffee and one for tea. The steps which are common for both are shown in the center line and those that vary for the two beverages are shown in their specific parts.
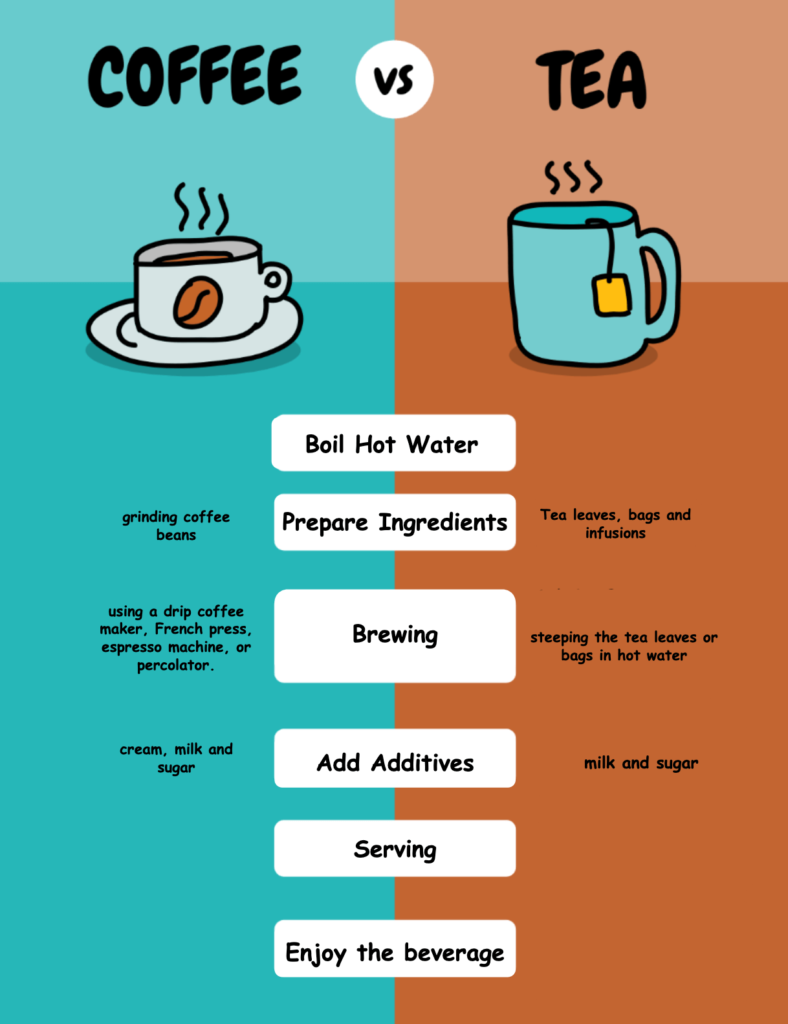
Structure of Template Method
The Template Method pattern involves the following key participants:
- Abstract Class (or Interface): This is the class that defines the template method, which is the skeleton of the algorithm. The template method consists of a series of steps, some of which may be implemented in the abstract class, while others are left to be implemented by concrete subclasses. It serves as a blueprint for the algorithm.
- Concrete Class (or Subclass): These are the classes that extend the abstract class and provide concrete implementations for the steps left open in the template method. Each concrete class represents a specific variation of the algorithm and customizes the behavior according to its requirements.
The interaction between these participants allows for the creation of a family of algorithms sharing a common structure, where subclasses can customize specific steps without altering the overall algorithmic framework. This promotes code reuse, encapsulation, and flexibility in adapting the algorithm to different scenarios.
Implementation of Template Method Pattern
Consider a program for generating different types of reports, such as CSV, HTML, and PDF. Each report follows a similar process but requires different implementations for certain steps.
Abstract Class ReportGenerator {
Method generateReport() {
data = collectData()
processedData = processData(data)
report = formatReport(processedData)
printReport(report)
}
Abstract Method collectData()
Abstract Method processData(data)
Abstract Method formatReport(processedData)
Method printReport(report) {
// Common implementation for printing the report
}
}
Class CSVReportGenerator extends ReportGenerator {
Method collectData() {
// Implementation for collecting CSV data
}
Method processData(data) {
// Implementation for processing CSV data
}
Method formatReport(processedData) {
// Implementation for formatting CSV report
}
}
Class HTMLReportGenerator extends ReportGenerator {
// Implement collectData, processData, and formatReport specifically for HTML
}
Class PDFReportGenerator extends ReportGenerator {
// Implement collectData, processData, and formatReport specifically for PDF
}
// Client Code
Main {
csvReportGenerator = new CSVReportGenerator()
csvReportGenerator.generateReport()
htmlReportGenerator = new HTMLReportGenerator()
htmlReportGenerator.generateReport()
pdfReportGenerator = new PDFReportGenerator()
pdfReportGenerator.generateReport()
}
- ReportGenerator (Abstract Class): Defines the skeleton of the report generation algorithm in the
generateReport
method. This method calls abstract methods (collectData
,processData
,formatReport
) and a concrete method (printReport
). - Concrete Classes (CSVReportGenerator, HTMLReportGenerator, PDFReportGenerator): Implement the abstract methods defined in
ReportGenerator
. Each class provides a specific implementation for collecting data, processing it, and formatting it according to the report type (CSV, HTML, or PDF). - printReport (Concrete Method): Provides a common implementation for printing the report, used by all subclasses.
This structure allows for defining the common steps of report generation in the base class while enabling subclasses to implement specific behavior for different report types. It reduces redundancy and allows for extending the system with new report types easily.
Implementation
Here’s an example of the Template Method Pattern in Java:
package com.ashok.designpatterns.template;
/**
*
* @author ashok.mariyala
*
*/
public abstract class ReportGenerator {
protected abstract String collectData();
protected abstract String processData(String data);
protected abstract String formatReport(String processedData);
private void printReport(String report) {
System.out.println(report);
}
public void generateReport() {
String data = collectData();
String processedData = processData(data);
String report = formatReport(processedData);
printReport(report);
}
}
class CSVReportGenerator extends ReportGenerator {
protected String collectData() {
return "CSV Data";
}
protected String processData(String data) {
return "Processed " + data;
}
protected String formatReport(String processedData) {
return "CSV Report: " + processedData;
}
}
// Similar classes for HTMLReportGenerator and PDFReportGenerator
public class Solution {
public static void main(String[] args) {
ReportGenerator csvReport = new CSVReportGenerator();
csvReport.generateReport();
// Similarly for other report types
}
}
Application of Template Method Pattern
Some notable applications of Template method pattern include:
1. Software Frameworks
Graphical User Interface (GUI) Frameworks: Template Method is often employed in GUI frameworks where the overall structure of handling user inputs and events is predefined, but the specific behavior may vary in different applications or components.
Web Frameworks: Web frameworks utilize Template Method to provide a structured approach for handling HTTP requests and responses. The overall request lifecycle remains consistent, but developers can customize specific steps based on application requirements.
2. Algorithm Design
Sorting Algorithms: Template Method can be applied to sorting algorithms. The overall sorting process remains the same, but specific steps like element comparison or swapping may vary depending on the sorting algorithm (e.g., quicksort, mergesort).
Search Algorithms: Similar to sorting, search algorithms can benefit from the Template Method pattern. The search process structure remains consistent, while specific steps like comparing elements may differ.
3. Game Development
Game Design Frameworks: In game development, the Template Method pattern can be employed in frameworks that define the overall game loop structure. While the main loop remains constant, specific behaviors for updating game objects or handling user input can be customized by individual games.
Character Animation: When designing character animation systems, a common structure for updating and rendering characters can be defined using the Template Method. The specifics of animation sequences can vary for different characters.
4. Educational Software
Learning Management Systems: Educational software often involves structured processes for presenting content, quizzes, and assessments. A Template Method can define the overall flow, while individual courses or modules provide specific implementations for content delivery and assessments.
5. Document Generation
Report Generation: In applications generating reports or documents, a Template Method can define the structure of document creation. Specific steps for adding headers, footers, and content generation can be customized based on the type of document.
6. Business Process Automation
Workflow Management Systems: Systems that automate business processes can benefit from the Template Method pattern. The overall workflow structure remains consistent, but specific steps for handling tasks, approvals, or notifications can be tailored to different business processes.
In summary, the Template Method pattern offers advantages in terms of code reuse, consistent structure, and flexibility for customization. However, it comes with trade-offs such as increased complexity, potential tight coupling, and limited runtime flexibility. Choosing to use the Template Method pattern should consider the specific requirements and design goals of the application.
The Template Method Pattern is highly useful for defining algorithms with a common structure but variable steps, promoting code reuse, consistency, and separation of concerns.
That’s all about the Template Method Pattern. If you have any queries or feedback, please write us email at contact@waytoeasylearn.com. Enjoy learning, Enjoy Design patterns.!!