Spring Boot Interview Questions
This tutorial would walk you through the Spring boot interview questions for freshers and experienced, scope, and opportunities.
Spring boot is the hottest topic of discussion in interviews if it comes to Java Application development. Because of its fast, low configuration, inbuild server, and monitoring features, it helps to build a stand-alone java application from scratch with very robust and maintainable.
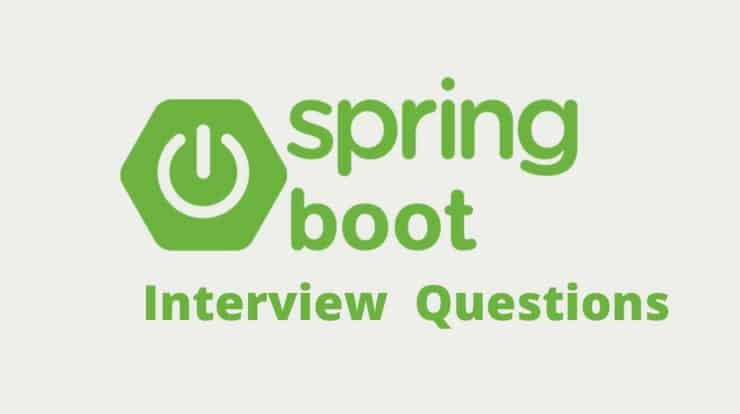
1. What is Spring Boot?
Spring Boot is a Spring module that provides RAD (Rapid Application Development) features to the Spring framework. It is used to create stand-alone Spring-based applications that you can just run. So, it basically removes a lot of configurations and dependencies.
So, if you ask me why should anybody use Spring Boot, then I would say, Spring Boot not only improves productivity but also provides a lot of conveniences to write your own business logic.
2. What are the Advantages of Spring Boot that make it different?
- Creates stand-alone spring application with minimal configuration needed.
- It has embedded tomcat, and jetty which makes it just code and run the application. You don’t need to deploy WAR files.
- Provide production-ready features such as metrics, health checks, and externalized configuration.
- Absolutely no requirement for XML configuration.
- Increases productivity and reduces development time.
- Comes with Spring Boot starters to ensure dependency management and also provides various security metrics
3. What is the difference between Spring and Spring Boot?
Spring | Spring Boot |
---|---|
Spring is an open-source lightweight framework widely used to develop enterprise applications. | Spring Boot is built on top of the conventional spring framework, widely used to develop REST APIs. |
The most important feature of the Spring Framework is dependency injection. | The most important feature of the Spring Boot is Autoconfiguration. |
To run the Spring application, we need to set the server explicitly. | Spring Boot provides embedded servers such as Tomcat and Jetty etc. |
To create a Spring application, the developers write lots of code. | It reduces the lines of code. |
It doesn’t provide support for the in-memory database. | It provides support for the in-memory database such as H2. |
A deployment descriptor is required for running Spring applications. | A deployment descriptor is not required for Spring Boot. |
Needs dependencies to be defined manually | Starters take care of dependencies |
4. Mention a few features of Spring Boot?
A few important features of Spring Boot are as follows:
- Spring CLI – Spring Boot CLI allows you to Groovy for writing Spring boot applications and avoids boilerplate code.
- Starter Dependency – With the help of this feature, Spring Boot aggregates common dependencies together and eventually improves productivity
- Auto-Configuration – The auto-configuration feature of Spring Boot helps in loading the default configurations according to the project you are working on. In this way, you can avoid any unnecessary WAR files.
- Spring Initializer – This is basically a web application, which can create an internal project structure for you. So, you do not have to manually set up the structure of the project, instead, you can use this feature.
- Spring Actuator – Spring boot’s actuator module allows us to monitor and manage application usages in a production environment, without coding and configuration for any of them. This monitoring and management information is exposed via REST like endpoint URLs.
- Logging and Security – The logging and security feature of Spring Boot, ensures that all the applications made using Spring Boot are properly secured without any hassle.
5. What are the Spring Boot key components?
Below are the four key components of spring-boot:
- Spring Boot auto-configuration.
- Spring Boot starter POMs.
- Spring Boot CLI.
- Spring Boot Actuators.
6. Why Spring Boot over Spring?
Below are some key points that spring boot offers but spring doesn’t.
- Starter POM.
- Version Management.
- Auto Configuration.
- Component Scanning.
- Embedded server.
- InMemory DB.
- Actuators
Spring Boot simplifies the spring feature for the user.
7. What is the starter dependency of the Spring boot module?
Spring boot provides a number of starter dependencies, here are the most commonly used.
- Data JPA starter.
- Test Starter.
- Security starter.
- Web starter.
- Mail starter.
- Thymeleaf starter.
- Spring AOP stater
- JDBC stater
8. How does Spring Boot work?
Spring Boot automatically configures your application based on the dependencies you have added to the project by using annotation. The entry point of the spring boot application is the class that contains the @SpringBootApplication annotation and the main method.
Spring Boot automatically scans all the components included in the project by using the @ComponentScan annotation.
9. What does the @SpringBootApplication annotation do internally?
The @SpringBootApplication annotation is equivalent to using @Configuration, @EnableAutoConfiguration, and @ComponentScan with their default attributes. Spring Boot enables the developer to use a single annotation instead of using multiple. But, as we know, Spring provided loosely coupled features that we can use for each annotation as per our project needs.
10. What is the purpose of using @ComponentScan in the class files?
Spring Boot application scans all the beans and package declarations when the application initializes. You need to add the @ComponentScan annotation for your class file to scan the components added to your project.
We can scan multiple packages by specifying the package names.
@ComponentScan({"com.ashok.spring.first","com.ashok.spring.second"})
11. How does a spring boot application get started?
Just like any other Java program, a Spring Boot application must have a main method. This method serves as an entry point, which invokes the SpringApplication#run method to bootstrap the application.
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class);
// other statements
}
}
12. What are starter dependencies?
Spring boot starter is a maven template that contains a collection of all the relevant transitive dependencies that are needed to start a particular functionality. Like we need to import spring-boot-starter-web dependency for creating a web application.
<dependency>
<groupId> org.springframework.boot</groupId>
<artifactId> spring-boot-starter-web </artifactId>
</dependency>
13. What is Spring Initializer?
Spring Initializer is a web application that helps you to create an initial spring boot project structure and provides a maven or Gradle file to build your code. It solves the problem of setting up a framework when you are starting a project from scratch.
14. What is Spring Boot CLI and what are its benefits?
The Spring Boot CLI is a Command Line Interface for Spring Boot. It can be used for a quick start with Spring. It can run Groovy scripts which means that a developer need not write boilerplate code; all that is needed is to focus on business logic. Spring Boot CLI is the fastest way to create a Spring-based application.
15. What Are the Basic Annotations that Spring Boot Offers?
@EnableAutoConfiguration – to make Spring Boot look for auto-configuration beans on its classpath and automatically apply them.
@SpringBootApplication – used to denote the main class of a Boot Application. This annotation combines @Configuration, @EnableAutoConfiguration, and @ComponentScan annotations with their default attributes.
16. What is Spring Boot dependency management?
Spring Boot dependency management is used to manage dependencies and configuration automatically without you specifying the version for any of that dependencies.
17. Can we create a non-web application in Spring Boot?
Yes, we can create a non-web application by removing the web dependencies from the classpath (Remove web stater dependency in pom.xml) along with changing the way Spring Boot creates the application context.
18. Is it possible to change the port of the embedded Tomcat server in Spring Boot?
Yes, it is possible. By using the server.port in the application.properties.
19. What is the default port of tomcat in spring boot?
The default port of the tomcat server-id 8080. It can be changed by adding sever.port properties in the application.properties file.
20. Can we override or replace the Embedded tomcat server in Spring Boot?
Yes, we can replace the Embedded Tomcat server with any server (Currently only Jetty and Undertow are supported) by using the Starter dependency in the pom.xml file. Like you can use spring-boot-starter-jetty as a dependency for using a jetty server in your project. First of all, you need to exclude tomcat:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<!-- Exclude the Tomcat dependency -->
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
Then you can add your desired embedded web server:
<!-- Jetty Embedded Web Server -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jetty</artifactId>
</dependency>
or
<!-- Undertow Embedded Web Server -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-undertow</artifactId>
</dependency>
21. Can we disable the default web server in the Spring boot application?
Spring Boot automatically starts an application in web server mode if it finds the web starter module in the classpath. To disable web server configuration, set the web application type to none in the application.properties file as in this example:
spring.main.web-application-type=none
22. How to disable a specific auto-configuration class?
You can use exclude attribute of @EnableAutoConfiguration if you want auto-configuration not to apply to any specific class.
@EnableAutoConfiguration(exclude={className})
E.g
@Configuration
@EnableAutoConfiguration(exclude = {DataSourceAutoConfiguration.class, DataSourceTransactionManagerAutoConfiguration.class, HibernateJpaAutoConfiguration.class})
public class ClientAppConfiguration {
//it can be left blank
}
23. Explain @RestController annotation in Sprint boot?
It is a combination of @Controller and @ResponseBody, used for creating a restful controller. It converts the response to JSON or XML. It ensures that data returned by each method will be written straight into the response body instead of returning a template.
24. What is the difference between @RestController and @Controller in Spring Boot?
The main difference between the @restcontroller and the @controller is the @restcontroller combination of the @controller and @ResponseBody annotation. RestController: RestController is used for making restful web services with the help of the @RestController annotation.
25. What is the difference between RequestMapping and GetMapping?
RequestMapping can be used with GET, POST, PUT, and many other request methods using the method attribute on the annotation. Whereas GetMapping is only an extension of RequestMapping which helps you to improve on clarity on requests.
26. What is the use of Profiles in Spring Boot?
While developing the application we deal with multiple environments such as dev, QA, and Production, and each environment requires a different configuration. For example, we might be using an embedded H2 database for dev but for prod, we might have proprietary Oracle or DB2. Even if DBMS is the same across the environment, the URLs will be different.
To make this easy and clean, Spring has the provision Profiles to keep the separate configuration of environments.
27. What is Spring Actuator? What are its advantages?
An actuator is an additional feature of Spring that helps you to monitor and manage your application when you push it to production. These actuators include auditing, health, CPU usage, HTTP hits, metric gathering, and many more that are automatically applied to your application.
28. How to enable Actuator in the Spring boot application?
To enable the spring actuator feature, we need to add the dependency of “spring-boot-starter-actuator” in pom.xml.
<dependency>
<groupId> org.springframework.boot</groupId>
<artifactId> spring-boot-starter-actuator </artifactId>
</dependency>
29. What are the actuator-provided endpoints used for monitoring the Spring boot application?
Actuators provide below pre-defined endpoints to monitor our application –
- Health
- Info
- Beans
- Mappings
- Configprops
- Httptrace
- Heapdump
- Thread dump
- Shutdown
30. How to get the list of all the beans in your Spring boot application?
Spring Boot actuator “/beans” is used to get the list of all the spring beans in your application.
31. How to check the environment properties in your Spring boot application?
Spring Boot actuator “/env” returns the list of all the environment properties of running the spring boot application.
32. How to enable debugging log in the spring boot application?
Debugging logs can be enabled in three ways –
- We can start the application with –debug switch.
- We can set the logging.level.root=debug property in application.properties file.
- We can set the logging level of the root logger to debug in the supplied logging configuration file.
33. Where do we define properties in the Spring Boot application?
You can define both application and Spring boot-related properties into a file called application.properties. You can create this file manually or use Spring Initializer to create this file. You don’t need to do any special configuration to instruct Spring Boot to load this file, If it exists in classpath then spring boot automatically loads it and configure itself and the application code accordingly.
34. What is dependency Injection?
The process of injecting dependent bean objects into target bean objects is called dependency injection.
- Setter Injection: The IOC container will inject the dependent bean object into the target bean object by calling the setter method.
- Constructor Injection: The IOC container will inject the dependent bean object into the target bean object by calling the target bean constructor.
35. What is an IOC container?
IoC Container is a framework for implementing automatic dependency injection. Spring IoC Container is the core of Spring Framework. It creates the objects, configures and assembles their dependencies, manages their entire life cycle.
36. Explain what is thymeleaf and how to use thymeleaf?
Thymeleaf is a server-side Java template engine used for web applications. It aims to bring natural template for your web application and can integrate well with Spring Framework and HTML5 Java web applications. To use Thymeleaf, you need to add the following code in the pom.xml file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
37. What is the need for Spring Boot DevTools?
Spring Boot 1.3 provides another module called Spring Boot DevTools. DevTools stands for Developer Tool. The aim of the module is to try and improve the development time while working with the Spring Boot application. Spring Boot DevTools pick up the changes and restart the application.
To include the DevTools, you just have to add the following dependency into the pom.xml file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
</dependency>
38. How can we create a custom endpoint in Spring Boot Actuator?
To create a custom actuator endpoints, Use @Endpoint annotation on a class. Then leverage @ReadOperation
/ @WriteOperation
/ @DeleteOperation
annotations on the methods to expose them as actuator endpoint bean as needed.
@Endpoint(id = "helloworld")
public class HelloWorldEndpoint {
}
@Configuration
public class CustomActuatorConfiguration {
@Bean
public HelloWorldEndpoint helloWorldEndpoint() {
return new HelloWorldEndpoint();
}
}
Lastly, before any of the endpoints we define in our HelloWorldEndpoint class are accessible we must tell Spring to expose our endpoint from its properties.
management.endpoints.web.exposure.include=helloworld
39. What is Spring Data?
Spring Data is a high level SpringSource project whose purpose is to unify and ease the access to different kinds of persistence stores, both relational database systems and NoSQL data stores.
With every kind of persistence store, your repositories typically offer CRUD operations on single domain objects, finder methods, sorting and pagination. Spring Data provides generic interfaces for these aspects (CrudRepository, PagingAndSortingRepository) as well as persistence store specific implementations.
40. Mention the advantages of the YAML file than Properties file and the different ways to load YAML file in Spring boot.
The advantages of the YAML file than a properties file is that the data is stored in a hierarchical format. So, it becomes very easy for the developers to debug if there is an issue. The SpringApplication class supports the YAML file as an alternative to properties whenever you use the SnakeYAML library on your classpath. The different ways to load a YAML file in Spring Boot is as follows:
- Use YamlMapFactoryBean to load YAML as a Map
- Use YamlPropertiesFactoryBean to load YAML as Properties