Type Casting in Python
In this tutorial, we are going to discuss about Type Casting in python programming language.
- We can convert one type value to another type. This conversion is called Typecasting or Type coercion.
- The following are various inbuilt functions for type casting.
int()
float()
complex()
bool()
str()
1. int()
We can use this function to convert values from other types to int.
>>> int(123.987)
123
>>> int(10+5j)
TypeError: can't convert complex to int
>>> int(True)
1
>>> int(False)
0
>>> int("10")
10
>>> int("10.5")
ValueError: invalid literal for int() with base 10: '10.5'
>>> int("ten")
ValueError: invalid literal for int() with base 10: 'ten'
>>> int("0B1111")
ValueError: invalid literal for int() with base 10: '0B1111'
Note
- We can convert from any type to int except complex type.
- If we want to convert str type to int type, compulsory str should contain only integral value and should be specified in base-10.
2. float()
We can use float() function to convert other type values to float type.
>>> float(10)
10.0
>>> float(10+5j)
TypeError: can't convert complex to float
>>> float(True)
1.0
>>> float(False)
0.0
>>> float("10")
10.0
>>> float("10.5")
10.5
>>> float("ten")
ValueError: could not convert string to float: 'ten'
>>> float("0B1111")
ValueError: could not convert string to float: '0B1111'
Note
- We can convert any type value to float type except complex type.
- Whenever we are trying to convert str type to float type compulsory str should be either integral or floating point literal and should be specified only in base-10.
3. complex()
We can use complex() function to convert other types to complex type.
Form-1: complex(x)
We can use this function to convert x into complex number with real part x and imaginary part 0.
complex(10)==>10+0j
complex(10.5)===>10.5+0j
complex(True)==>1+0j
complex(False)==>0j
complex("10")==>10+0j
complex("10.5")==>10.5+0j
complex("ten")
ValueError: complex() arg is a malformed string
Form-2: complex(x,y)
We can use this method to convert x and y into complex number such that x will be real part and y will be imaginary part.
E.g
complex(10, -2) ==> 10-2j
complex(True, False) ==> 1+0j
4. bool()
We can use this function to convert other type values to bool type.
bool(0) ==> False
bool(1) ==> True
bool(10) ==> True
bool(10.5) ==> True
bool(0.178) ==> True
bool(0.0) ==> False
bool(10-2j) ==> True
bool(0+1.5j) ==> True
bool(0+0j) ==> False
bool("True") ==> True
bool("False") ==> True
bool("") ==> False
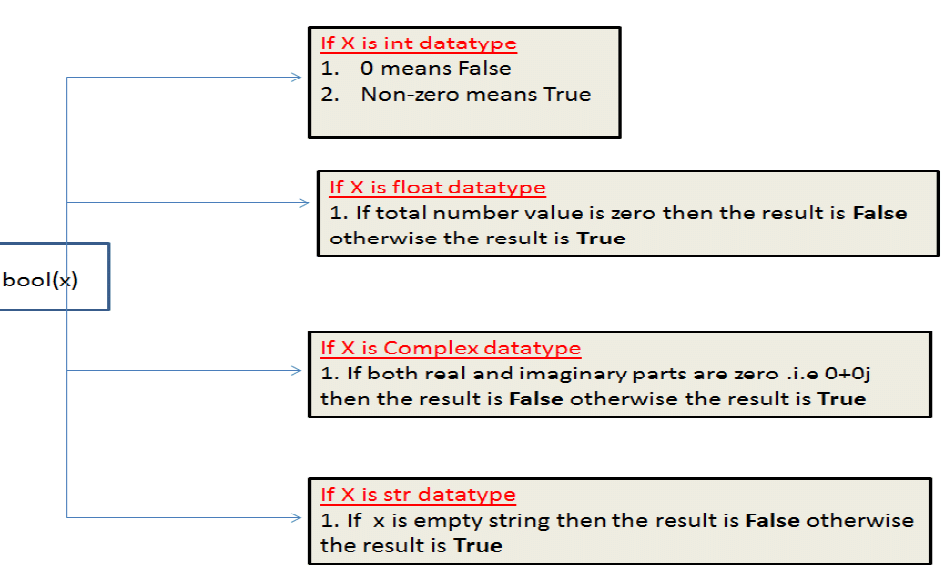
5. str()
We can use this method to convert other type values to str type.
>>> str(10)
'10'
>>> str(10.5)
'10.5'
>>> str(10+5j)
'(10+5j)'
>>> str(True)
'True'
Fundamental Data Types vs Immutability
All Fundamental Data types are immutable. i.e once we creates an object,we cannot perform any changes in that object. If we are trying to change then with those changes a new object will be created. This non-changeable behavior is called immutability.
In Python if a new object is required, then PVM won’t create object immediately. First it will check is any object available with the required content or not. If available then existing object will be reused. If it is not available then only a new object will be created. The advantage of this approach is memory utilization and performance will be improved.
But the problem in this approach is, several references pointing to the same object, by using one reference if we are allowed to change the content in the existing object then the remaining references will be effected. To prevent this immutability concept is required. According to this once creates an object we are not allowed to change content. If we are trying to change with those changes a new object will be created.
>>> a=10
>>> b=10
>>> a is b
True
>>> id(a)
1572353952
>>> id(b)
1572353952
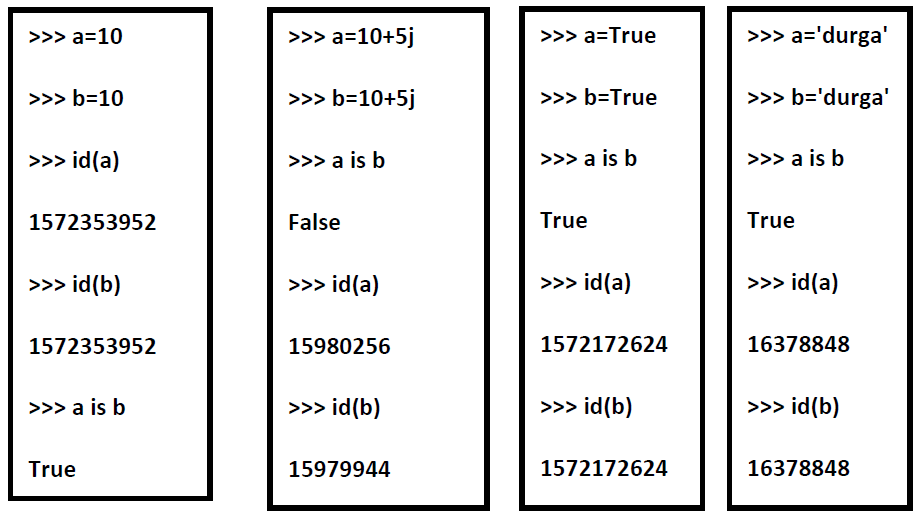
That’s all about the Type Casting in Python programming language. If you have any queries or feedback, please write us email at contact@waytoeasylearn.com. Enjoy learning, Enjoy Python language.!!