Vert.x JsonObject
In this tutorial, we are going to discuss about the Vert.x JsonObject. In Vert.x, a JsonObject
is a data structure that represents a JSON object, which consists of key-value pairs. It is used to handle and manipulate JSON data in a convenient way, often for messaging, configuration, or web API requests and responses. Vert.x provides JsonObject
as part of its core API to simplify working with JSON data in Java.
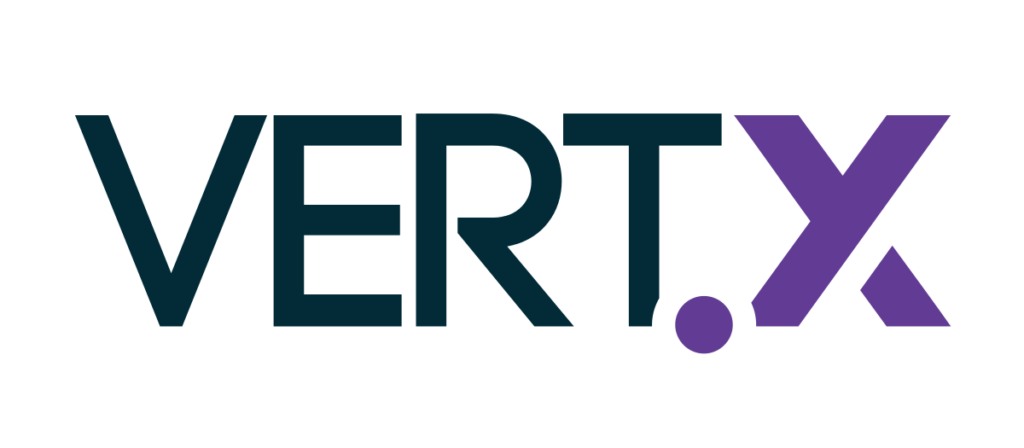
Vert.x JsonObject
is a lightweight, immutable representation of a JSON object, similar to how JSON objects work in JavaScript. It is commonly used for message passing, configuration settings, and structured data exchange across different verticles or components in Vert.x applications.
When working with web applications. Json is the de-facto standard. The majority of REST APIs use it. It is human readable and very flexible. Java does not have a Json object class out of the box, but Vert.x does. Vertex has a built in JsonObject
and JsonArray
implementation. It is possible to convert Java objects to the vertex Json object or send them over the event bus.
Vert.x JSON Object available in io.vertx.core.json.JsonObject
package.
Key Features of JsonObject
- Simple Key-Value Structure: Allows mapping between string keys and values.
- Immutable: Once created, its content is immutable, meaning any modifications result in a new object.
- Common Use Cases: Configuration, data passing over the event bus, HTTP requests/responses, and service interaction.
Creating A JsonObject
You can create a JsonObject
in multiple ways, including constructing it directly from a String
, adding key-value pairs manually, or converting other data types (like Maps) into JSON.
1. Create from Scratch
JsonObject jsonObject = new JsonObject();
jsonObject.put("name", "Ashok Kumar");
jsonObject.put("age", 32);
jsonObject.put("city", "Hyderabad");
System.out.println(jsonObject.encodePrettily());
Output:
{
"name": "Alice",
"age": 30,
"city": "New York"
}
2. Create from a JSON String
You can parse a JSON string directly into a JsonObject
.
String jsonString = "{\"name\": \"Ashok Kumar\", \"age\": 32, \"city\": \"Hyderabad\"}";
JsonObject jsonObject = new JsonObject(jsonString);
System.out.println(jsonObject.encodePrettily());
3. Create from a Map
If you already have a Map
in Java, you can easily convert it into a JsonObject
.
Map<String, Object> map = new HashMap<>();
map.put("name", "Ashok Kumar");
map.put("age", 32);
map.put("city", "Hyderabad");
JsonObject jsonObject = new JsonObject(map);
System.out.println(jsonObject.encodePrettily());
Accessing Values in a JsonObject
Once a JsonObject
is created, you can retrieve the values using various get
methods, depending on the data type of the value.
1. Retrieve String Value
String name = jsonObject.getString("name");
System.out.println("Name: " + name);
2. Retrieve Integer Value
Integer age = jsonObject.getInteger("age");
System.out.println("Age: " + age);
3. Retrieve Nested JsonObject
JsonObject jsonObject = new JsonObject();
JsonObject address = new JsonObject().put("street", "5th Avenue").put("city", "Hyderabad");
jsonObject.put("address", address);
JsonObject retrievedAddress = jsonObject.getJsonObject("address");
System.out.println(retrievedAddress.encodePrettily());
Output
{
"street": "5th Avenue",
"city": "Hyderabad"
}
Encoding JsonObject
You can convert a JsonObject
to a JSON string using either encode()
or encodePrettily()
.
encode()
: Produces a compact string representation of the JSON object.encodePrettily()
: Produces a more readable (pretty-printed) string representation.
String compactJson = jsonObject.encode(); // Compact
String prettyJson = jsonObject.encodePrettily(); // Pretty printed
Example
package com.ashok.vertx.vertx_starter.jsonobject;
import io.vertx.core.json.JsonObject;
/**
*
* @author ashok.mariyala
*
*/
public class JsonObjectExample {
public static void main(String[] args) {
JsonObject jsonObject = new JsonObject();
jsonObject.put("name", "Ashok Kumar");
jsonObject.put("age", 32);
jsonObject.put("city", "Hyderabad");
System.out.println(jsonObject.encode());
System.out.println(jsonObject.encodePrettily());
}
}
Output
{"name":"Ashok Kumar","age":32,"city":"Hyderabad"}
{
"name" : "Ashok Kumar",
"age" : 32,
"city" : "Hyderabad"
}
Handling Arrays with JsonObject
A Vert.x JsonObject can also contain arrays using the JsonArray class in Vert.x. Here’s how you can work with JSON arrays
Example
{
"name" : "Ashok Kumar",
"age" : 32,
"city" : "Hyderabad",
"hobbies" : [ "Reading", "Traveling", "Cooking" ]
}
Accessing JsonArray
Values
package com.ashok.vertx.vertx_starter.jsonobject;
import io.vertx.core.json.JsonArray;
import io.vertx.core.json.JsonObject;
/**
*
* @author ashok.mariyala
*
*/
public class JsonObjectExample {
public static void main(String[] args) {
JsonObject jsonObject = new JsonObject();
jsonObject.put("name", "Ashok Kumar");
jsonObject.put("age", 32);
jsonObject.put("city", "Hyderabad");
JsonArray hobbies = new JsonArray().add("Reading").add("Traveling").add("Cooking");
jsonObject.put("hobbies", hobbies);
JsonArray hobbiesArray = jsonObject.getJsonArray("hobbies");
String firstHobby = hobbiesArray.getString(0);
System.out.println("First Hobby: " + firstHobby);
}
}
Output
First Hobby: Reading
Combining JsonObject
and Event Bus
Vert.x JsonObject
is often used when sending or receiving messages on the Vert.x Event Bus because JSON is a common format for exchanging data between services.
Sending JsonObject
on Event Bus
JsonObject message = new JsonObject().put("name", "Alice").put("age", 30);
vertx.eventBus().send("address", message);
Receiving JsonObject
on Event Bus
vertx.eventBus().consumer("address", message -> {
JsonObject receivedMessage = (JsonObject) message.body();
System.out.println("Received: " + receivedMessage.encodePrettily());
});
Summary
- Vert.x
JsonObject
is a flexible, easy-to-use data structure that closely mirrors JSON objects. - It supports complex, nested data structures and is often used for passing data across the Vert.x event bus, HTTP servers/clients, or between verticles.
- Encoding and decoding JSON is simple and supports both compact and pretty-printed formats.
- Vert.x
JsonObject
integrates seamlessly with other Vert.x components like event bus and HTTP servers. - Vert.x JsonObject provides methods to create, update, and access JSON-like data using a simple API.
That’s all about the Vert.x JsonObject with examples. If you have any queries or feedback, please write us email at contact@waytoeasylearn.com. Enjoy learning, Enjoy Vert.x tutorials..!!