Handle NULLs in SQL
In this tutorial, we are going to discuss about how to handle NULLs in SQL. Handling NULL
values in SQL is essential to ensure data integrity and accurate query results. NULL
represents a missing or unknown value, and it can behave differently from other values in SQL.
What are Null Values?
Null values in MySQL indicate the absence of a value in a column. Unlike an empty string or zero, null is not a valid data value and typically represents missing or unknown information. Columns in a MySQL table can be defined to allow or disallow null values based on the data requirements.
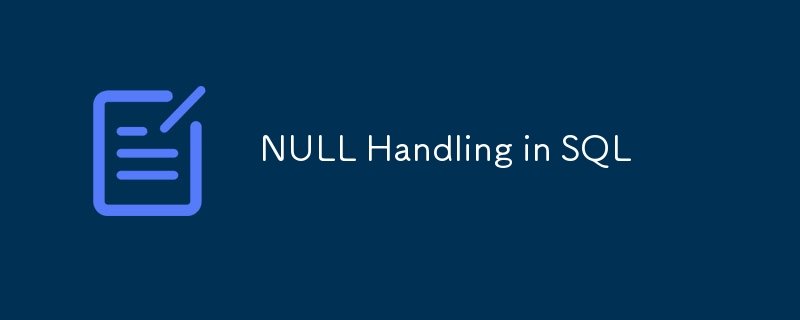
Here are some common methods to handle NULLs
:
Handling Null Values
1. Allowing Null Values in Columns:
When defining a table, you can specify whether a column allows null values or not. This is done using the NULL
attribute in the column definition.
CREATE TABLE students (
id INT,
email VARCHAR(255) NULL,
name VARCHAR(255)
);
In the above example, email
allows null values, while name
does not.
2. Checking for Null Values:
To check if a column contains null values, you can use the IS NULL
or IS NOT NULL
condition in a WHERE clause.
SELECT * FROM students WHERE email IS NULL;
This query retrieves rows where email
contains null values.
3. Using IS NULL and IS NOT NULL to Check for NULL
To filter records based on whether a column is NULL
or not:
SELECT *
FROM table_name
WHERE column_name IS NULL;
SELECT *
FROM table_name
WHERE column_name IS NOT NULL;
4. Using COALESCE
to Replace NULL
COALESCE returns the first non-NULL value in a list:
SELECT COALESCE(column_name, 'default_value') AS column_name
FROM table_name;
5. Using IFNULL
or NVL
to Replace NULL
These functions provide similar functionality to COALESCE
but are specific to certain SQL databases:
1. MySQL
SELECT IFNULL(column_name, 'default_value') AS column_name
FROM table_name;
2. Oracle
SELECT NVL(column_name, 'default_value') AS column_name
FROM table_name;
6. Using CASE Statements
CASE
can provide more complex conditional logic:
SELECT
CASE
WHEN column_name IS NULL THEN 'default_value'
ELSE column_name
END AS column_name
FROM table_name;
7. Handling NULL in Aggregations
Aggregations like SUM
, AVG
, MAX
, etc., automatically ignore NULL
values. To include NULL
values, you can use COALESCE
or similar functions:
SELECT SUM(COALESCE(column_name, 0)) AS total
FROM table_name;
8. NULL-safe Comparisons
- MySQL supports the
<=>
operator forNULL
-safe comparisons:
SELECT *
FROM table_name
WHERE column_name <=> NULL;
9. Sorting with NULLS FIRST or NULLS LAST
Some databases support sorting options for NULL
values:
SELECT *
FROM table_name
ORDER BY column_name ASC NULLS LAST;
These techniques help manage NULL
values efficiently, ensuring they don’t lead to unexpected results in your queries.
That’s all about the how to Handle NULLs in SQL. If you have any queries or feedback, please write us at contact@waytoeasylearn.com. Enjoy learning, Enjoy SQL..!!