Supplier Functional Interface
Sometimes our requirement is we have to get some value based on some operation like Supply Student object
Supply Random Name
Supply Random OTP
Supply Random Password etc
For this type of requirements we should go for Supplier. Supplier can be used to supply items (objects). Supplier won’t take any input and it will always supply objects. Supplier Functional Interface contains only one method get().
interface Supplier<R> { public R get(); }
Supplier Functional interface does not contain any default and static methods.
Supplier to generate Random Name
package com.ashok.java8.supplier; import java.util.function.Supplier; /** * * @author ashok.mariyala * */ public class SupplierTest { public static void main(String[] args) { Supplier<String> s = () -> { String[] str = { "Ashok", "Vinod", "Ajay", "Akshara", "Kumari" }; int x = (int) (Math.random() * 5); return str[x]; }; System.out.println(s.get()); System.out.println(s.get()); System.out.println(s.get()); System.out.println(s.get()); } }
Output
Kumari Akshara Vinod Kumari
Supplier to supply 6-digit Random OTP
package com.ashok.java8.supplier; import java.util.function.Supplier; /** * * @author ashok.mariyala * */ public class RandomOTP { public static void main(String[] args) { Supplier<String> otps = () -> { String otp = ""; for (int i = 1; i <= 6; i++) { otp = otp + (int) (Math.random() * 10); } return otp; }; System.out.println(otps.get()); System.out.println(otps.get()); System.out.println(otps.get()); System.out.println(otps.get()); } }
Output
954228 690936 248961 281114
Comparison Table of Predicate, Function, Consumer and Supplier
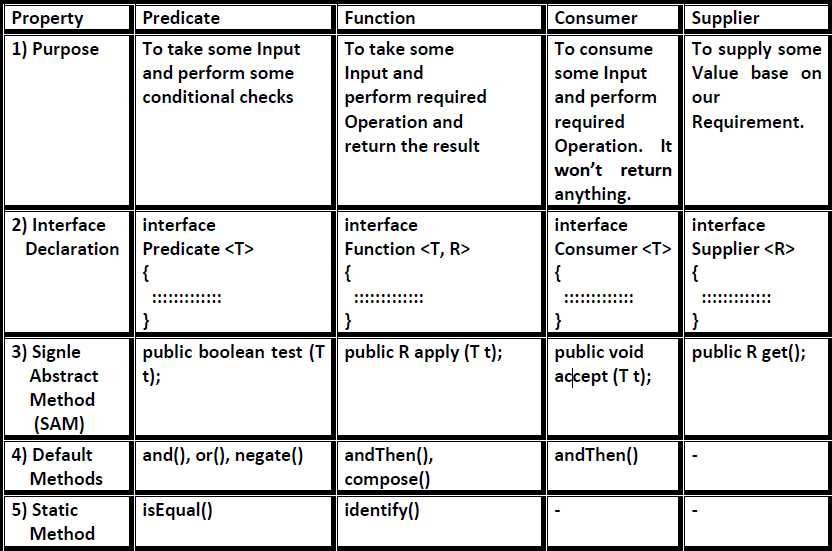
Supplier Functional Interface